Do you find it very tedious to type in file paths in Python?
By using tkinter, you can read file paths by simply selecting files with the mouse!
This article explains how to select multiple files from Explorer and get their file paths in GUI format.
Explain all functions of tkinter.filedialog
tkinter.filedialog handles file relationships in a GUI format.
- Parameters
-
- parent : the window to place the dialog on top of
- title : the title of the window
- initialdir : the directory that the dialog starts in
- initialfile : the file selected upon opening of the dialog
- filetypes : a sequence of (label, pattern) tuples, ‘*’ wildcard is allowed
- defaultextension : default extension to append to file (save dialogs)
- multiple : when true, selection of multiple items is allowed
- Functions
-
- tkinter.filedialog.askopenfile : Get a single file object(Open dialog)
- tkinter.filedialog.askopenfiles : Get multiple file objects(Open dialog)
- tkinter.filedialog.asksaveasfile : Get a single file object(SaveAs dialog)
- tkinter.filedialog.askopenfilename : Get a single file path(Open dialog)
- tkinter.filedialog.askopenfilenames : Get multiple file paths(Open dialog)
- tkinter.filedialog.asksaveasfilename : Get a single file path(SaveAs dialog)
- tkinter.filedialog.askdirectory : Get a single folder path
- Official Documentation
Get a single file path with askopenfilename (title, initialdir)
askopenfilename
can get the selected file path as a string
However, only one file can be selected.
Also, title (window title) and initialdir (starting directory) are specified as arguments.
path = filedialog.askopenfilename(title='Useful-Python', initialdir='C:/Users/Documents/')
print(path)
# C:/Users/Documents/230430_person_info_west.csv
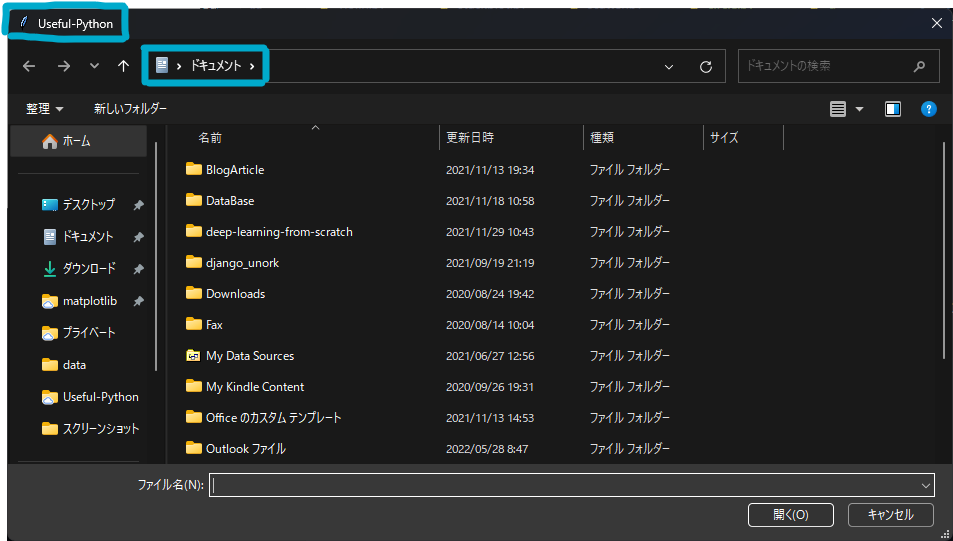
Get multiple file paths with askopenfilenames (initialfile, filetypes)
askopenfilenames
allows you to get the selected file paths as an array (tuple) of strings
It also takes two arguments: initialfile (initially selected file) and filetypes (selectable file types).
path = filedialog.askopenfilenames(
initialfile='230430_person_info_west.csv',
filetypes=[('CSVファイル', '*.csv')]
)
print(path)
# ('C:/Users/Documents/230430_person_info_west.csv',)
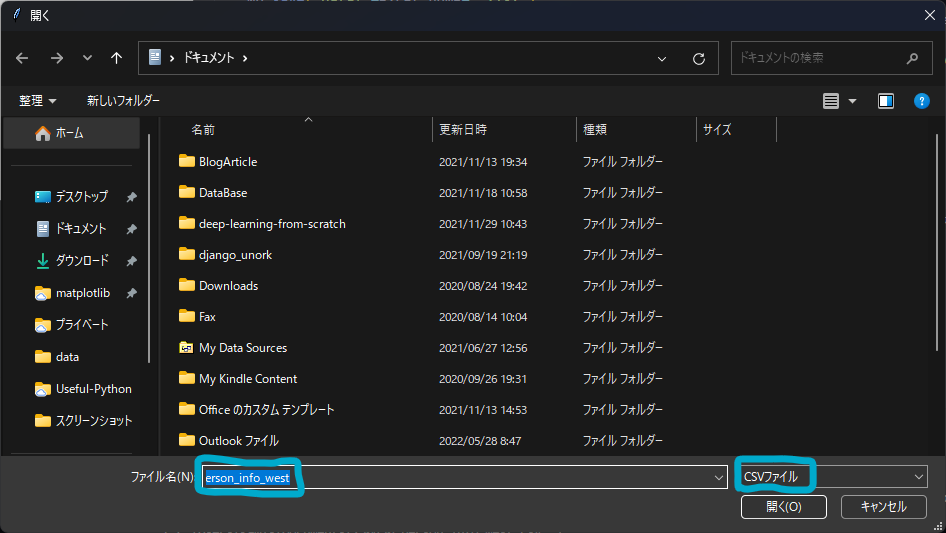
Get a single folder path in askdirectory
askdirectory
can get the selected folder path as a string
path = filedialog.askdirectory()
print(path)
# C:/Users/Documents
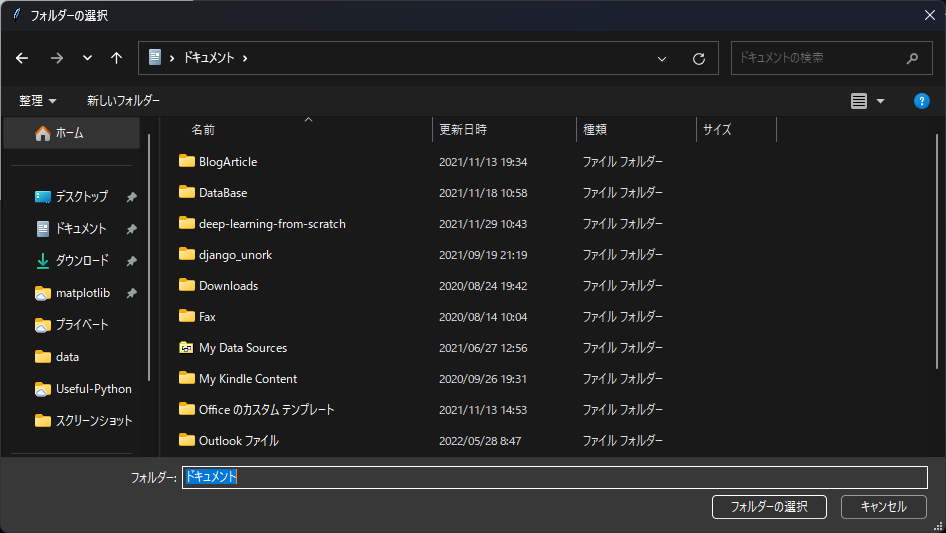
Make it a function and use it many times
Since the function to retrieve a file path is used in all kinds of situations, let’s make it a function that can be used just by calling it!
Function to get multiple file paths
The following code is a function to get multiple file paths with askopenfilenames.
The function name is read_filepathes
def read_filepathes() -> tuple:
typ = [('CSV,Text File', '*.csv; *.txt')]
dir = r'C:'
return filedialog.askopenfilenames(filetypes=typ, initialdir=dir)
Execute function
The function is executed in read_filepathes()
and the return value specified in return is assigned to pathes
This is exactly the array of file paths
pathes = read_filepathes()
print(pathes)
# ('C:/Users/Documents/230430_person_info_west.csv',)
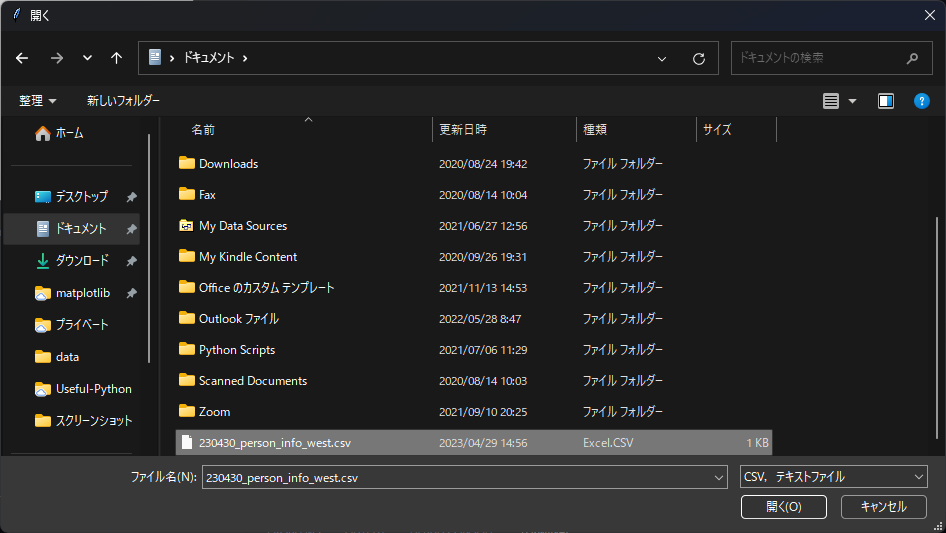
References
Official documentation for Tkinter Dialogs
Comments