Have you ever used Anaconda on a regular basis and wanted to try web app development?
In this article, I will build a django environment for web app development with Anaconda.
The target OS is Windows 64bit.
Please refer to the following article to learn how to build an environment combining Anaconda and Visual Studio Code.
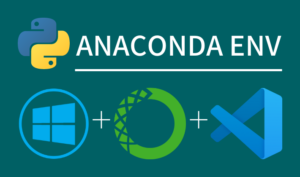
Build a virtual environment with Anaconda
First, let’s set up the Anaconda environment
Start command prompt and create a django virtual environment
conda create -n django_book python=3.8
File name: “django_book”
Python version: 3.8
After the virtual environment has been successfully created, activate it from the command prompt.
After activating, check the python version
conda activate django_book
python -V
# Python 3.8.11
Install django in a virtual environment
Next, we’ll put django into the anaconda virtual environment.
In this case, the virtual environment will be called django_book
Let’s search for django in Anaconda org and see if it exists
conda install -c conda-forge django
Modules installed in anaconda can be seen in the conda list
conda list
# Name Version Build Channel
asgiref 3.4.1 pyhd8ed1ab_0 conda-forge
ca-certificates 2021.5.30 h5b45459_0 conda-forge
certifi 2021.5.30 py38haa244fe_0 conda-forge
django 3.2.7 pyhd8ed1ab_0 conda-forge
openssl 1.1.1l h8ffe710_0 conda-forge
pip 21.0.1 py38haa95532_0
python 3.8.11 h6244533_1
python_abi 3.8 2_cp38 conda-forge
pytz 2021.1 pyhd8ed1ab_0 conda-forge
setuptools 58.0.4 py38haa95532_0
sqlite 3.36.0 h2bbff1b_0
sqlparse 0.4.2 pyhd8ed1ab_0 conda-forge
typing_extensions 3.10.0.2 pyha770c72_0 conda-forge
vc 14.2 h21ff451_1
vs2015_runtime 14.27.29016 h5e58377_2
wheel 0.37.0 pyhd3eb1b0_1
wincertstore 0.2 py38haa95532_2
I was able to get django in.
Create a django project
Now let’s start a django project
First, go to your working folder
cd Django/Getting_Start
From here on, the procedure is the same as the official one
Create a project named django_project
django-admin startproject django_project
This should create a django_project in the current folder
django_project/
manage.py
django_project/
__init__.py
settings.py
urls.py
asgi.py
wsgi.py
Once the project is created, add the application to django
The name of the application this time is “blog”.
cd django_project
python manage.py startapp blog
This will generate a blog folder
blog/
__init__.py
admin.py
apps.py
migrations/
__init__.py
models.py
tests.py
urls.py
views.py
Create the HTML and create what you want to display on the screen
Write the following code in blog/views.py
from django.http import HttpResponse
def index(request):
return HttpResponse("Hi, World")
Next, create urls.py in the blog folder
Once created, write the following code in blog/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
And also register the url of the app in django_project/urls.py
from django.contrib import admin
from django.urls import path, include #Add
urlpatterns = [
path('', include('blog.urls')), #Add
path('admin/', admin.site.urls),
]
Start the django project and complete the site
Finally, start django
Start django from command prompt
python manage.py runserver
Click on the url displayed in the command prompt to access it!
http://127.0.0.1:8000/
Comments