We often want to visualize data in real-time, for example, to monitor numerical experiments or to see how numerical data from machine learning or deep learning is improving.
In this article, we will explain how to plot graphs in real time using Matplotlib in python.
It is implemented using the pause and remove functions.
In addition to the simple implementation, I will also show you how to add a legend and how to change the color of the graph to rainbow.
Easily plot graphs in real time (pause)
Real-time graphs in Matplotlib use the function pyplot.pause.
- pause(float)
-
If a number is specified in parentheses, it stops for that number of seconds.
- Examples
-
plt.pause(0.001)
This article plots a line graph by appending to a list of x, y1, y2.
import numpy as np
import matplotlib.pyplot as plt
# step1 Create data
xs = []
y1 = []
y2 = []
# step2 Create graph frames
fig, ax = plt.subplots()
# step3 Update graphs in real time
for x in np.linspace(0, 10, 100):
# Update values
xs.append(x)
y1.append(4 + 2 * np.sin(2 * x))
y2.append(4 + 2 * np.cos(2 * x))
# Plot graphs
ax.plot(xs, y1, color='C0', linestyle='-')
ax.plot(xs, y2, color='C1', linestyle='--')
ax.set_xlabel('X label')
ax.set_ylabel('Y label')
# Stop for 0.001 second
plt.pause(0.001)
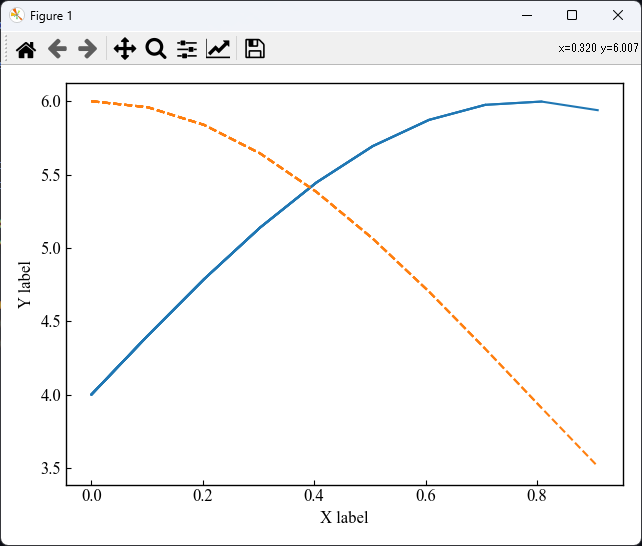
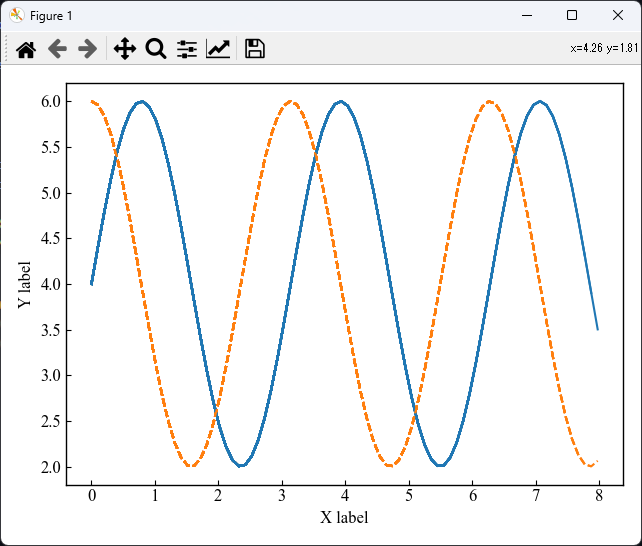
Plot graphs in real time with legends (remove)
The legend of the real-time graph must be deleted from the graph each time.
Then Axes.remove function removes the graph.
- Axes object.remove()
-
rIf a graph object created with ax.plot is connected with a dot just before remove, it will be removed.
- Examples
-
line1, = ax.plot(xs, y1)
line1.remove()
# step3 Update graphs in real time
for x in np.linspace(0, 10, 100):
# Update values
xs.append(x)
y1.append(4 + 2 * np.sin(2 * x))
y2.append(4 + 2 * np.cos(2 * x))
# Plot graphs
line1, = ax.plot(xs, y1, color='C0', linestyle='-', label='Sample1')
line2, = ax.plot(xs, y2, color='C1', linestyle='--', label='Sample2')
# Stop for 0.001 second
plt.pause(0.001)
# Delete plotted graphs
line1.remove()
line2.remove()
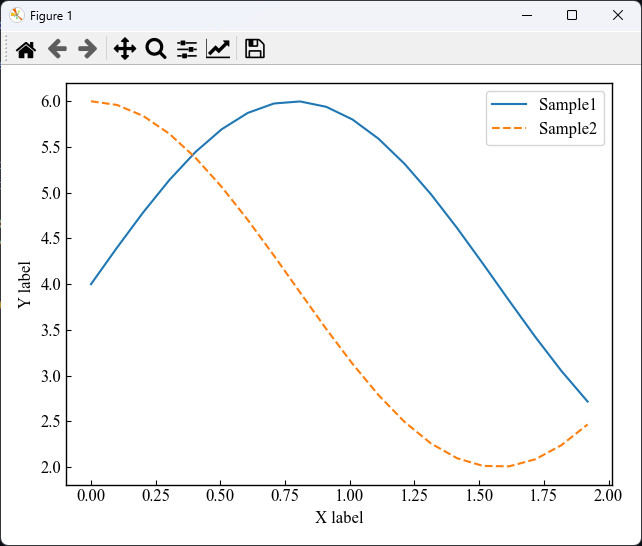
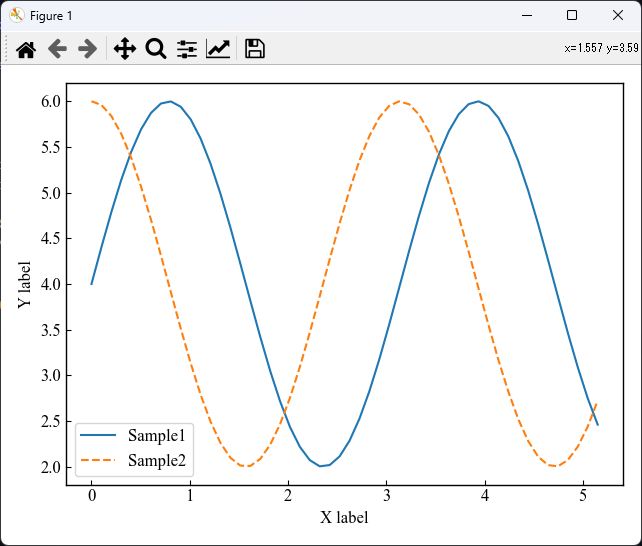
Rainbow the color of the graph in real time
If you do not specify a color, the line will switch infinitely and the line color will be rainbow.
Please try it out with the code below!
xs = []
y1 = []
y2 = []
fig, ax = plt.subplots()
for x in np.linspace(0, 10, 100):
xs.append(x)
y1.append(4 + 2 * np.sin(2 * x))
y2.append(4 + 2 * np.cos(2 * x))
line1, = ax.plot(xs, y1, linestyle='-', label='Sample1')
line2, = ax.plot(xs, y2, linestyle='--', label='Sample2')
plt.pause(0.001)
line1.remove()
line2.remove()
References
Official documentation of the pause function
Official documentation of the remove function
Comments